When we work DevOps pipelines, pipeline logs often help us diagnose what went wrong or right. An easy way to make text stand out is to put some colors. For example, red text instantly directs our attention to errors in a haystack of text.
Observation: Console.ForegroundColor does not work
Let me just start with an observation that an intuitive approach does not work, and here is the proof 😉
// render the default font
Console.WriteLine("Normal style");
// the standard C# way: it works in console, but not in DevOps pipelines
Console.ForegroundColor = ConsoleColor.Cyan;
Console.WriteLine("Console.ForegroundColor = ConsoleColor.Cyan");
Console.BackgroundColor = ConsoleColor.Yellow;
Console.WriteLine("Console.BackgroundColor = ConsoleColor.Yellow");
Console.ResetColor();
Code language: C# (cs)

Option 1: use Logging Commands
In Azure DevOps, one way to write text in color is to use Logging Commands:
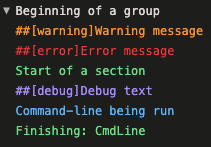
Logging Commands are useful for highlighting specific log sections with relevant semantic meanings. However, using these commands may lead to unintended side effects, such as warnings appearing in other areas of the DevOps UI, which may be undesirable at times. If you want to write some log line in color, but not give it any semantic meaning, check the next option.
Option 2: use ANSI escape codes
We can also use ANSI escape codes. Well, maybe not all of them. In general, based on my observations, DevOps seems to currently support escape sequences that set foreground or background color and nothing more—but this is what we need here 🙂
namespace WriteSomethingInColor;
internal class Program
{
static void Main(string[] args)
{
// Preview foreground colors
for (int fontColor = 30; fontColor <= 37; fontColor++)
Console.WriteLine($"\u001b[{fontColor}m example (foreground color {fontColor})\u001b[0m");
// Preview background colors
Console.WriteLine();
for (int fontColor = 40; fontColor <= 47; fontColor++)
Console.WriteLine($"\u001b[{fontColor}m example (background color {fontColor})\u001b[0m");
// Prove that we can mix custom font and background color
Console.WriteLine();
Console.WriteLine($"\u001b[46m\u001b[33ma yellow font on a blue background\u001b[0m");
Console.WriteLine($"\u001b[47m\u001b[31ma red font on a white background\u001b[0m");
Console.WriteLine("\u001b[0m... and now the color is reset back to the default value");
}
}
Code language: C# (cs)
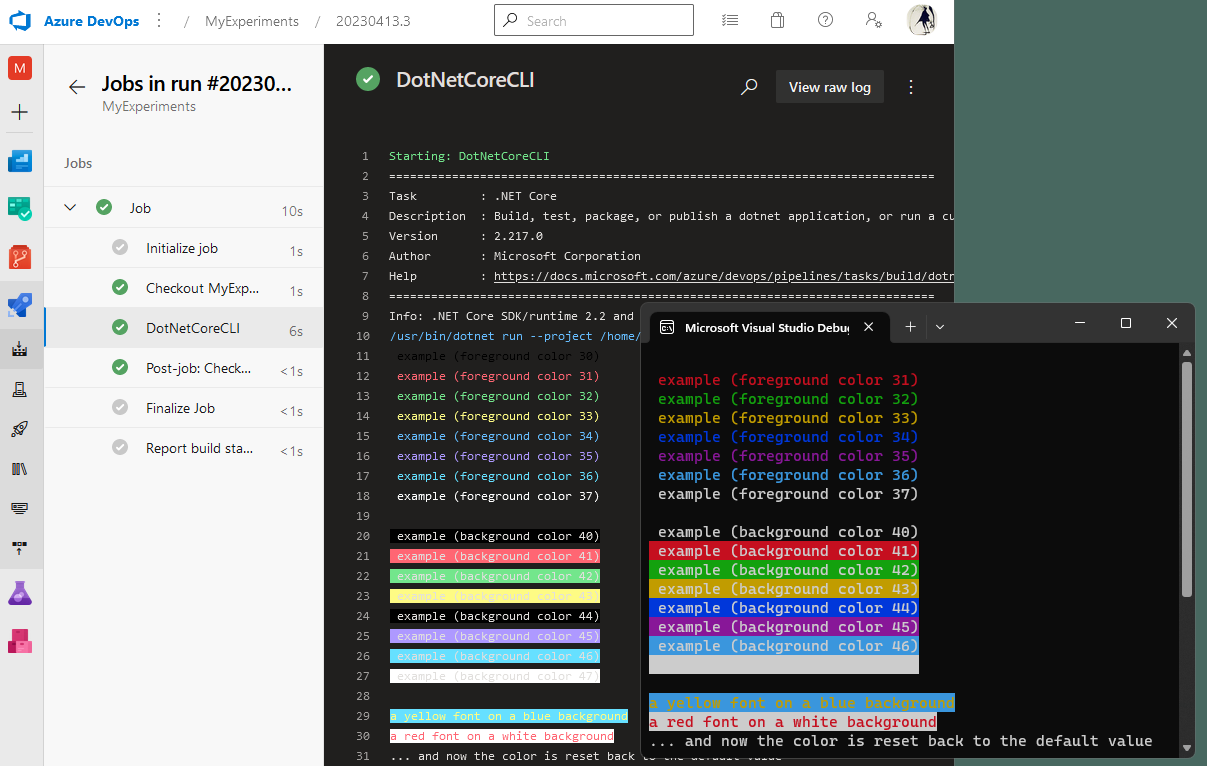
The shades of colors rendered in DevOps and in Windows command line with default settings are close enough. I think we can expect that color 32 will be rendered as green in all environments, for example.
Hi,
Thanks for this usefull information.
I try it on Framework 6.0, and it works.
But I am on old legacy 4.6.2 .Net Framework, and It does not work…
Any idea of workaround to be able to do the same with 4.6.2 ?
Thanks for advance.
Regards
Jp
Hi Jean-Pierre,
Thanks for the comment! I tried to reproduce the problem, but it works just fine on my side in .NET Framework 4.6.2.
Did you find the source of the issue already?
I refreshed this article a bit for clarity.
The new code sample is tested to work in both .NET 7 and .NET Framework 4.6.2. Both in Windows console and in DevOps pipelines context.