.NET 7 introduced the [GeneratedRegex]
attribute that allows doing some regex initialization in compile time rather than in runtime. Using this attribute might help improve performance. Therefore some tooling might start showing you a warning that you miss an opportunity for free performance gains 🙂
SYSLIB1045 Use ‘GeneratedRegexAttribute’ to generate the regular expression implementation at compile-time.
Since the documentation is scarce at the moment, I’ll share a simple example of how you can resolve that warning from my own experience:
Code before the change
// OLD IMPLEMENTATION
private static readonly Regex MultipleWhitespacesRegex = new(@"\s+");
private string OldImplementation(string input) => MultipleWhitespacesRegex.Replace(input, " ");
Code language: C# (cs)
Code after the recommended change
// NEW IMPLEMENTATION
[GeneratedRegex(@"\s+")]
private static partial Regex MultipleWhitespacesGeneratedRegex();
private string NewImplementation(string input) => MultipleWhitespacesGeneratedRegex().Replace(input, " ");
// please note, the class where this field is located must also now be declared as partial!
Code language: C# (cs)
A quick benchmark
Even though I changed this only to resolve warnings in the codebase, I couldn’t resist seeing if there was a noticeable performance difference when the new attribute was used. And although my regular expression was extremely simple, the new approach still performed slightly better:
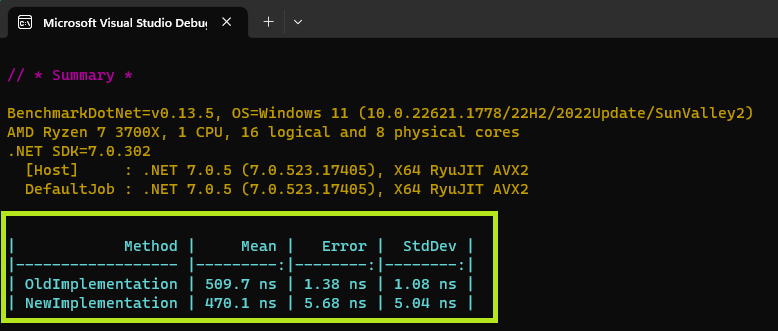
As always, when we talk about performance, results may vary. You should not assume that something is faster just because it should be, etc. You know the drill 😉
No comments yet, you can leave the first one!