I like to maintain a zero-warning policy in a project. In the case of false-alarm warnings, I think it’s better to suppress them than to leave them active. When we suppress a warning, it will not draw our attention every time we look at logs.
So, how can we suppress a warning like the example one below?
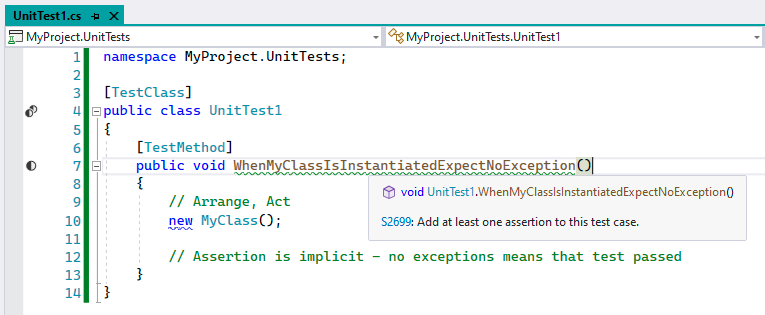
Option 1: pragma directives
Visual Studio suggests that method by default. I find it ugly because it decreases the readability of the code. Especially that in the default code style rules, the code has no indentation before the pragma directive.
[TestMethod]
#pragma warning disable S2699 // Tests should include assertions
public void WhenMyClassIsInstantiatedExpectNoException()
#pragma warning restore S2699 // Tests should include assertions
{
// Arrange, Act
new MyClass();
// Assertion is implicit. No exceptions means that the test passed.
}
Code language: C# (cs)
Option 2: the SuppressMessage attribute
I believe that the System.Diagnostics.CodeAnalysis.SuppressMessage attribute leads to much more elegant code. It doesn’t decrease readability that much when we scan the code with our eyes:
[TestMethod]
[SuppressMessage("Blocker Code Smell", "S2699:Tests should include assertions",
Justification = "Assertion is implicit. No exceptions means that the test passed.")]
public void WhenMyClassIsInstantiatedExpectNoException()
{
new MyClass();
}
Code language: C# (cs)
The only piece of that attribute which is really required is the second argument, with the code of a suppressed warning. This abbreviated form would technically work equally well:
[SuppressMessage("Sonar", "S2699")]
Code language: C# (cs)
Option 3: exclusions in *.csproj
If we want to globally disable some warnings in the scope of the entire project, the *.csproj file is an excellent place to do so. We can specify multiple warnings, separating them with a comma or a semicolon.
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>net6.0</TargetFramework>
<Nullable>enable</Nullable>
<NoWarn>S2699</NoWarn>
</PropertyGroup>
<!-- ... the rest of the file -->
</Project>
Code language: HTML, XML (xml)
Note: Using Directory.Build.props for project-wide configuration
For cases where you want to apply suppression across multiple projects in a solution, you could use a Directory.Build.props file. This allows you to centralize standard configurations, such as suppressing warnings:
<Project>
<PropertyGroup>
<!-- Don't warn about the need for .ConfigureAwait(false) CA2007 in console app projects -->
<NoWarn>$(NoWarn);CA2007</NoWarn>
</PropertyGroup>
</Project>
Code language: HTML, XML (xml)
This approach is particularly useful for solutions with consistent warning suppression policies across multiple projects.
Option 4: the .editorconfig file
The severity of the warnings, including the ones from the custom code analyzers like SonarAnalyzer, can also be configured in the .editorconfig file. Visual Studio 2022 even has a nice UI for that:
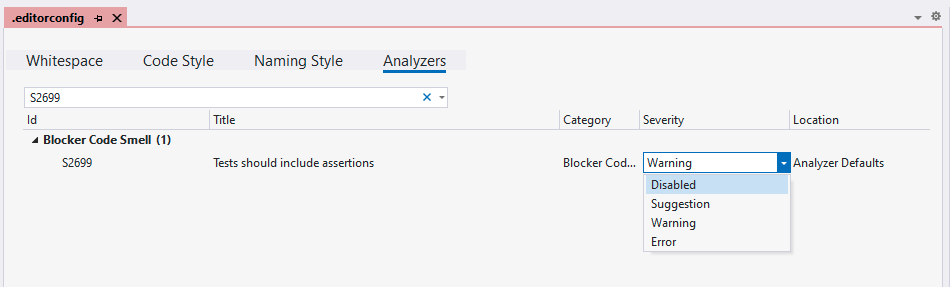
Under the hood it’s still just a plain text file, and the setting translates to the following fragment in the .editorconfig file:
[*.cs]
dotnet_diagnostic.S2699.severity = none
Option 5: a suppression file
A list of exceptions can also be centrally managed in a suppression file. I don’t see many advantages to this method. From my experience, in the projects it often grows into a pile of unmaintained rubbish. There is no natural incentive to ever delete anything from this file. It’s usually generated by the IDE and has some custom conventions for Target that we might not understand at first glance.
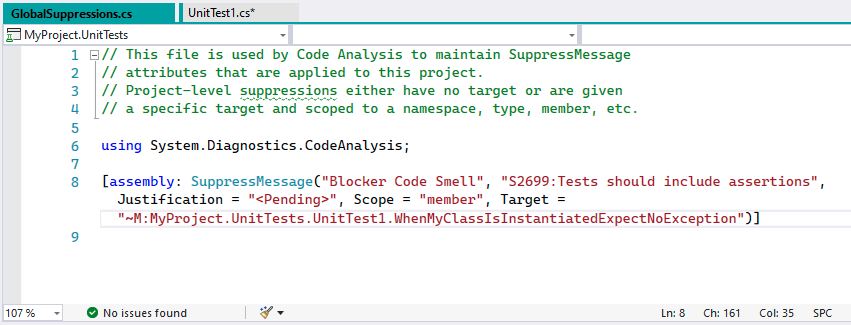
Have I missed any other option worth mentioning? 😉
Your article has been of great help to me, it helped me disable some warnings that do not affect the rest of my code in any way. Thank you very much for the information you provide.
I’m glad it was useful 🙂 Thanks for the kind words and good luck with your project!
You should also add Directory.Build.props. F.ex:
$(NoWarn);CA2007
Thanks for pointing that out Trygve! I integrated your suggestion into the `Option 3 (…)` section 🙂